Excel VBAコース(6):Excel VBA言語基本 - 変数
この記事では、Excel VBA言語の基本的な変数の内容について説明します。
前の記事で続く内容だ。
Excel VBAコース(5):Excelファイル拡張子、VBE、フォント設定
3. Excel VBA言語の基本
3.1。変数
変数宣言とbinding方式を見てみましょう。
3.1.1.変数宣言
次の構文で変数を宣言します。
'변수 선언 문법 Dim <변수명> [As Type]
Visual Basicは変数を宣言せずにすぐに使用でき、宣言してもTypeを指定しない可能性があります。
Dim t, x
上記のようにt変数とx変数を宣言し、Typeを指定しなくても問題はありません。
しかし、エラーやバグの発生の可能性を減らし、コードを読みやすくするために、次のことを守ることをお勧めします。
- 変数は必ず宣言して使用する。 (Option Explicit 推奨)
- Typeも必ず指定する。
- 変数名には、typeを簡単に理解できるように、typeの略語をprefixとして使用するハンガリアン表記法を適用する。
'문자열 Title Dim sTitle As String
- 変数名、プロシージャ名、関数名などの名称にハングルを使っても全く問題がないので、変数名を定めにくくしたり、読みやすさを高める必要があればハングル名称を積極的に使う。
主に使用するtype、prefixは次のとおりです。
Prefix | タイプ | 長さ | メモ |
---|---|---|---|
s | String | 10+文字列長 | 約20億個の文字表現可能 |
i | Integer | 2 Byte | 限界値:-32,768〜32,767 |
l | 長い | 4 Byte | 限界値:-2,147,483,648〜2,147,483,647 |
b | Boolean | 2 Byte | 真(True)、偽(False) |
o | Object | Workbook、Worksheet、Range、Collectionなどのオブジェクト |
上記のPrefixを使用して変数を宣言する例は次のとおりです。
Dim s단어 As String Dim b단어발견 As Boolean Dim oTargetBook As Workbook Dim oTargetSht As Worksheet
3.1.2。変数 Early binding, Late binding
変数typeが宣言されたときに決まる方法をEarly bindingと呼び、実行するときに決まる方法をLate bindingという。 2つの方法の概念、例、推奨事項、利点、欠点を次の表にまとめた。
区分 | Early binding | Late binding |
---|---|---|
コンセプト | 変数宣言時にTypeを指定する方法 組み込みタイプでない場合は、そのタイプを参照を追加してタイプを指定する | 実行時にTypeを指定する方法 |
例 | Dim s 単語 As String Dim oRecordSet As ADO.RecordSet | Dim s単語 Dim oRecordSet As Object |
推奨事項 | ほとんどの場合推奨 | 必ず必要な場合のみ使用 (Object typeのみ限定) |
利点 | コード作成時のオートコンプリート、動的ヘルプサポート(便利) コンパイル時にTypeが決定されるため、パフォーマンスに有利です。 | 実行しているコンピュータに参照ライブラリがない場合、Late bindingコード部分のみが実行されない |
欠点 | 実行しているコンピュータに参照ライブラリがないと、全体が実行されない | コード作成時にオートコンプリートがサポートされない(不便) 実行時にTypeが決定され、動的にビンディングされるため、パフォーマンスが比較的悪い |
各方法のサンプルコードを見てみましょう。 Outlookでメールの受信者、件名、内容を作成し、画面に表示する簡単なコードです。
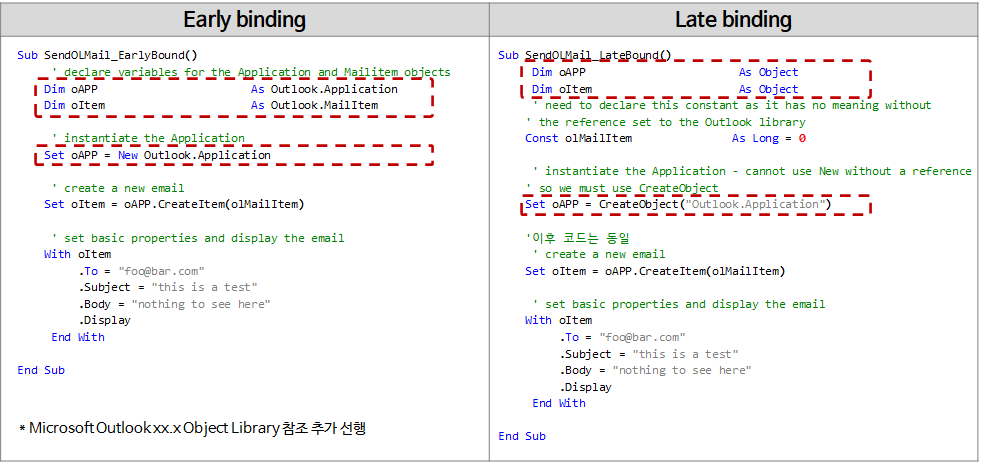
Early binding方式の例示コードは次の通りである。
Sub SendOLMail_EarlyBound() ' declare variables for the Application and Mailitem objects Dim oAPP As Outlook.Application Dim oItem As Outlook.MailItem ' instantiate the Application Set oAPP = New Outlook.Application ' create a new email Set oItem = oAPP.CreateItem(olMailItem) ' set basic properties and display the email With oItem .To = "foo@bar.com" .Subject = "this is a test" .Body = "nothing to see here" .Display End With End Sub
oApp、oItem変数はそれぞれOutlook.Application type、Outlook.MailItem typeとして宣言時に指定された。この場合は、次のようにOutlookライブラリを参照して追加する必要があります。
「VBE>ツール>参照」メニューを開き、「利用可能な参照」の「Microsoft Outlook 16.0 Object Library」にチェックマークを付けて、OKボタンをクリックします。
もう一度参照メニューを開いたときは、次のようになります。
このVBAコードが格納されているマクロファイル(.xlsm、または.xlsb)をOutlook 16がインストールされていないPCにコピーして実行すると、typeが見つからないというエラーが発生します。
Late binding方式の例示コードは次の通りである。
Sub SendOLMail_LateBound() Dim oAPP As Object Dim oItem As Object ' need to declare this constant as it has no meaning without ' the reference set to the Outlook library Const olMailItem As Long = 0 ' instantiate the Application - cannot use New without a reference ' so we must use CreateObject Set oAPP = CreateObject("Outlook.Application") '이후 코드는 동일 ' create a new email Set oItem = oAPP.CreateItem(olMailItem) ' set basic properties and display the email With oItem .To = "foo@bar.com" .Subject = "this is a test" .Body = "nothing to see here" .Display End With End Sub
oApp、oItem変数は宣言時にObject typeとして指定されており、オブジェクトの生成方法が異なります。
10行目の次のコードでオブジェクトを作成します。
Set oAPP = CreateObject(“Outlook.Application”)
CreateObject関数の詳細な説明は、次のURLを参照してください。
上記のURLから一部を抜粋して残しておく。
Syntax
CreateObject(class, [ servername ])
The CreateObject function syntax has these parts:
Part | Description |
クラス | Required; Variant (String)。 The application name and class of the object to create. |
servername | Optional; Variant (String)。 The name of the network server where the object will be created. If servername is an empty string (“”), the local machine is used. |
The class argument uses the syntax appname.objecttype and has these parts:
Part | Description |
appname | Required; Variant (String)。 The name of the application providing the object. |
objecttype | Required; Variant (String)。 The type or クラス of object to create. |
このVBAコードが格納されているマクロファイル(.xlsm、または.xlsb)をOutlook 16がインストールされていないPCにコピーして実行すると、Early bindingと同様にtypeが見つからないというエラーが発生します。違いは、Early binding方式は参照ライブラリがないと最初からすべてのコードが実行されず、Late bindingは他のコードは動作し、CreateObjectを含むコード(プロシージャ、関数)だけ実行されないという点だ。
ここまで変数宣言とbinding方式について見てきました。次に、VBA言語の文法(Syntax)について説明します。
<<関連記事一覧>>
- Excel VBAコースを開始します。 (講座予告、feat.Excel VBAを推奨する理由)
- Excel VBAコース(1):Excel VBAの概要
- Excel VBAコース(2):Excel VBAの基礎
- Excel VBAコース(3):Excel Object Model
- Excel VBAコース(4):Excel Object Modelを扱う
- Excel VBAコース(5):Excelファイル拡張子、VBE、フォント設定
- Excel VBAコース(6):Excel VBA言語基本 - 変数
- Excel VBAコース(7):Excel VBA言語基本 - 文法(Syntax)
- Excel VBAコース(8):Excel VBA言語基本 - データ型(Data type)、データ構造(Data structure)
- Excel VBAコース(9):Excel VBA How-To
- Excel VBAコース(10):Excel VBAで開発され使用されているツール
- Excel VBAコース全体目次